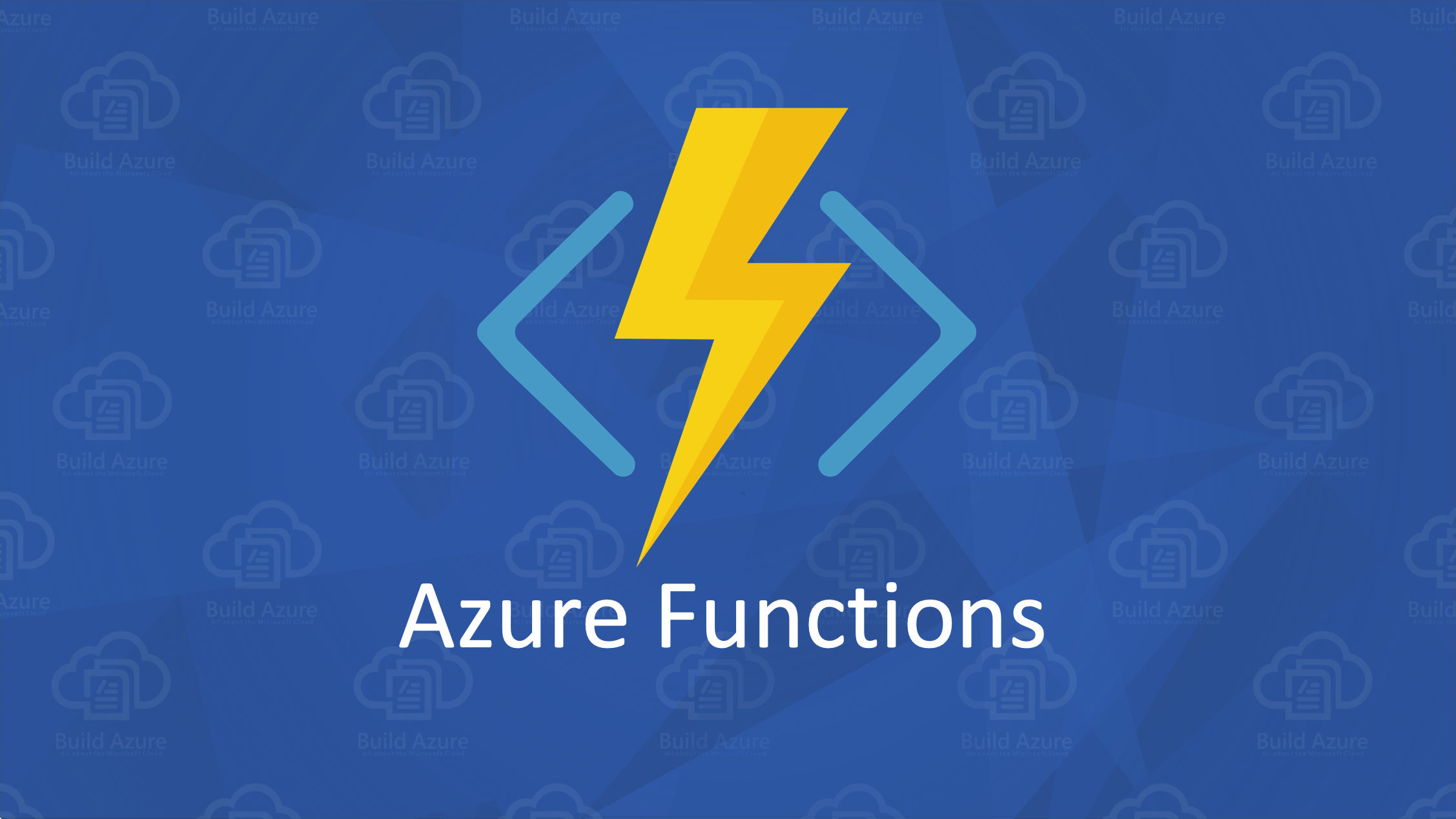
This article provides step-by-step instructions on how to access SharePoint data from Azure Functions using the Graph API.
Create a new Azure Active Directory App Registration
This is a common step when we need to access the Graph API from external systems.
Depending on the type of resource you’re accessing in your Azure function, you can choose API permissions, and you can choose between delegated or application permissions. In this particular case we choose Application Permissions and Site.ReadWrite.All.
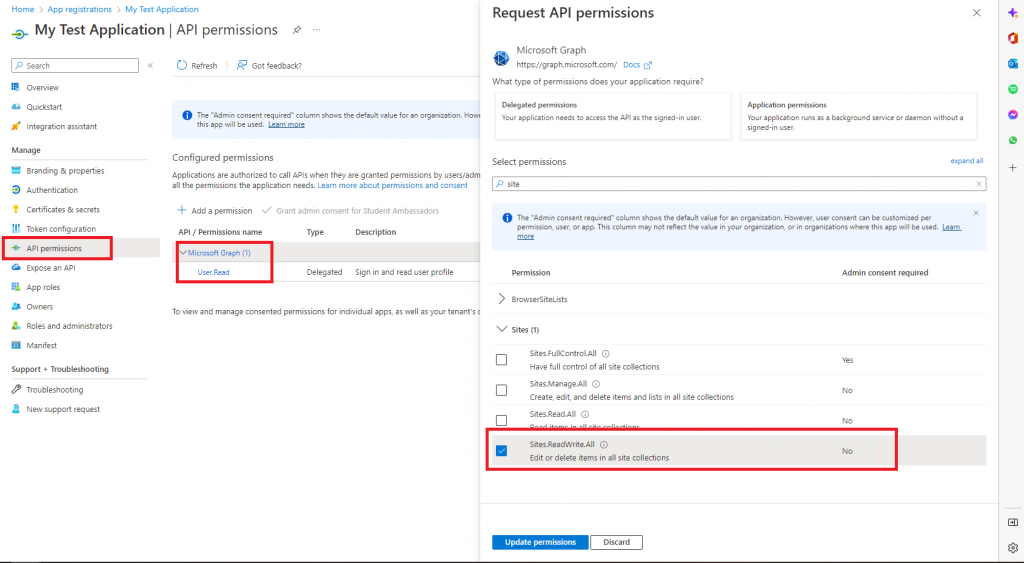
Grant admin consent as we will use application permissions and directly access SharePoint data without any user intervention. In this step, you need to write down the ClientId, Client Secret and Tenant ID, which will be used later.
Create Azure Function Project
We will now create a C#.net based Azure Function, but you can also use other supported languages to create.
Open Visual Studio (no code) – create a new project and select Azure Functions. You can check this link as well: Develop Azure Functions using Visual Studio | Microsoft Learn
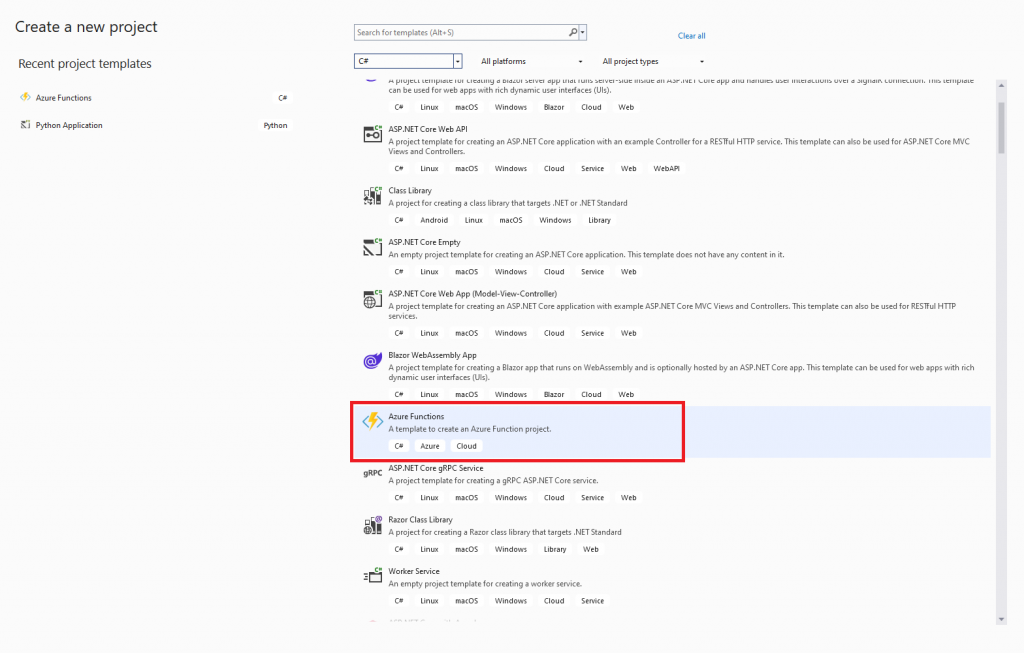
Choose your project name and solution path, go next and it will trigger type and .NET version. Choose Azure Functions Worker, Function (Http Trigger) and Authorization level.
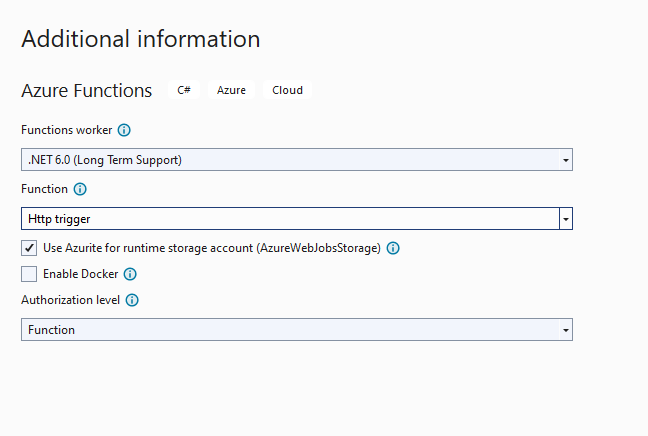
You can rename the function name and File name according to your preference. After that you can see the project like that:
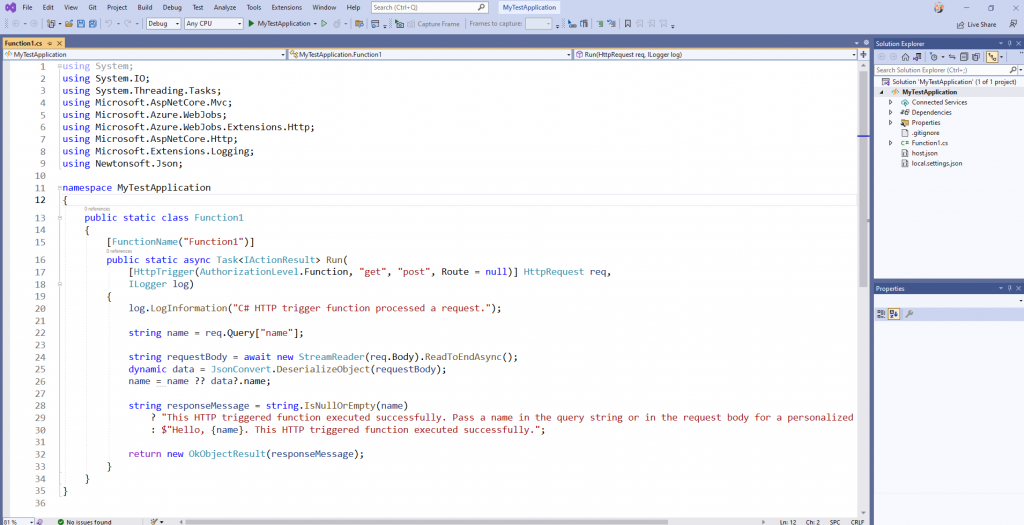
Because the purpose of this article is to obtain/access data from SharePoint. Let us begin by creating the files/functions/methods needed for authentication and the methods that will be used later for your business logic.
Install Graph SDK NuGet Packages
We’ll be using the NuGet Graph SDK package, which includes methods/functions and classes that can interact with any Graph endpoint and resource.
Navigate to Tools-> NuGet Package Manager – NuGet Packages for Solutions.
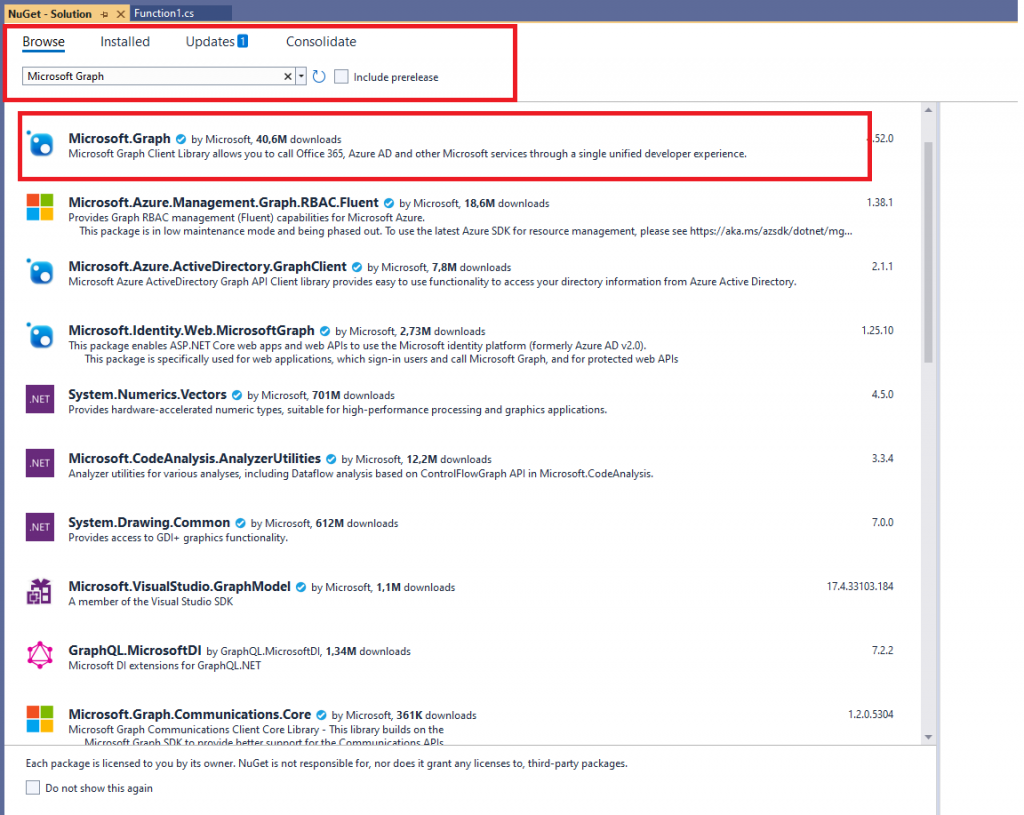
Click on Browse and search for Microsoft.Graph and install the libraries
Microsoft.Graph and a then similar way for Microsoft.Graph.Auth, Microsoft.Identity.Client
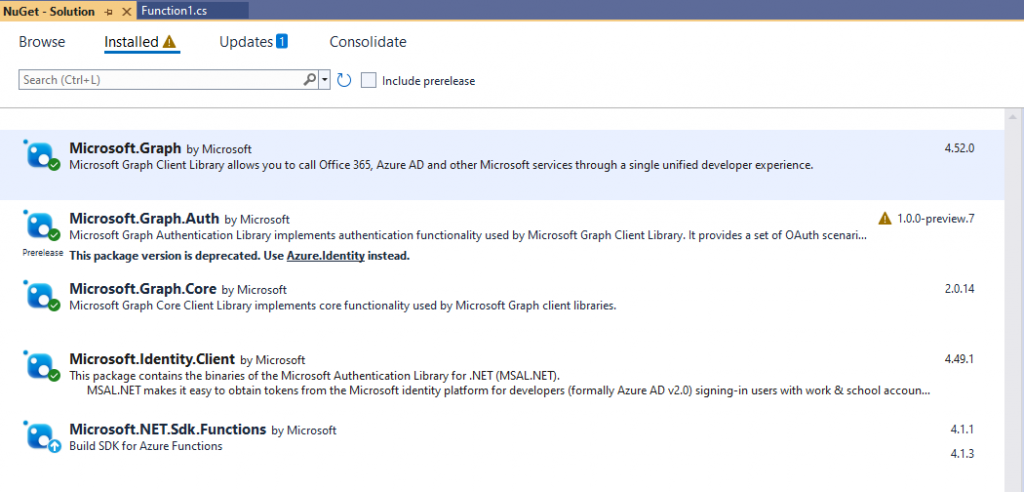
Once you’ve installed all of them. It is now time to begin writing and modifying code as needed.
Create a local configuration file, modify local.settings.json
{
"IsEncrypted": false,
"Values": {
"AzureWebJobsStorage": "UseDevelopmentStorage=true",
"FUNCTIONS_WORKER_RUNTIME": "dotnet",
"clientid": "App Registration Client Id",
"tenantid": "Your Tenant ID",
"clientSecret": "4123123123213123123adsfsadfsadf",
"siteId": "This is the Site ID to which you have to connect where the list is created but it is a little tricker, you can get this from Graph Explorer.",
"clientsListID": " ID of list from where we wanted to read data"
}
}
Go to Graph Explorer and hit the below endpoint and you will get Site ID in the above format.
https://graph.microsoft.com/v1.0/sites?search=NameofYoursite
Modify code to Read data from SP list and then write to the console
In an ideal world, you would process this list data, perform some operations, and return the data in JSON format, but for the sake of simplicity, we are simply writing data to the console and returning the default message that the HTTP Trigger function was successfully executed.
Below is high-level steps that are done in code
- To obtain configuration values such as client Id, secret, tenant Id, list Id, and site Id, read Environment Variable. When making an actual graph API call to get data from a list, this would be required.
- To connect to Graph Service, create a GraphServiceClient object with the client id, tenant id, and secret.
- To request items from the SP list, use the GraphServiceClient method.
- Write to the console after looping through the items returned.
You can copy the code and replace it with your code as well
using System;
using System.IO;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
using Microsoft.Graph;
using Microsoft.Identity.Client;
using Microsoft.Graph.Auth;
using System.Collections.Generic;
namespace MyTestApplication
{
public static class Function1
{
[FunctionName("Function1")]
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Function, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
string name = req.Query["name"];
string requestBody = await new StreamReader(req.Body).ReadToEndAsync();
dynamic data = JsonConvert.DeserializeObject(requestBody);
name = name ?? data?.name;
ConfigurationsValues configValues = readEnviornmentVariable();
GraphServiceClient _graphServiceClient = getGraphClient(configValues);
var queryOptions = new List<QueryOption>()
{
new QueryOption("expand", "fields(select=ID,Title,Name)")
};
IListItemsCollectionPage _listItems;
try
{
_listItems = await _graphServiceClient.Sites[configValues.SiteId].Lists[configValues.clientsListID].Items
.Request(queryOptions)
.GetAsync();
}
catch (Exception ex)
{
throw ex;
}
foreach (var item in _listItems.CurrentPage)
{
Console.WriteLine("-----------------------------Item ID " + item.Id+ "---------------------");
Console.WriteLine("Title---" + item.Fields.AdditionalData["Title"]);
Console.WriteLine("Name---" + item.Fields.AdditionalData["Name"]);
}
return new OkObjectResult("This HTTP triggered function executed successfully");
}
public static ConfigurationsValues readEnviornmentVariable()
{
ConfigurationsValues configValues = new ConfigurationsValues();
configValues.Tenantid = System.Environment.GetEnvironmentVariable("tenantid", EnvironmentVariableTarget.Process);
configValues.Clientid = System.Environment.GetEnvironmentVariable("clientid", EnvironmentVariableTarget.Process);
configValues.ClientSecret = System.Environment.GetEnvironmentVariable("clientsecret", EnvironmentVariableTarget.Process);
configValues.SiteId = System.Environment.GetEnvironmentVariable("siteId", EnvironmentVariableTarget.Process);
configValues.clientsListID = System.Environment.GetEnvironmentVariable("clientsListID", EnvironmentVariableTarget.Process);
return configValues;
}
public static GraphServiceClient getGraphClient(ConfigurationsValues configValues)
{
IConfidentialClientApplication confidentialClientApplication = ConfidentialClientApplicationBuilder
.Create(configValues.Clientid)
.WithTenantId(configValues.Tenantid)
.WithClientSecret(configValues.ClientSecret)
.Build();
ClientCredentialProvider authProvider = new ClientCredentialProvider(confidentialClientApplication);
GraphServiceClient graphClient = new GraphServiceClient(authProvider);
return graphClient;
}
}
public class ConfigurationsValues
{
public string Clientid { get; set; }
public string Tenantid { get; set; }
public string ClientSecret { get; set; }
public string SiteId { get; set; }
public string TargetedListId { get; set; }
public string clientsListID { get; set; }
}
}
Run the project and you can see the screen:
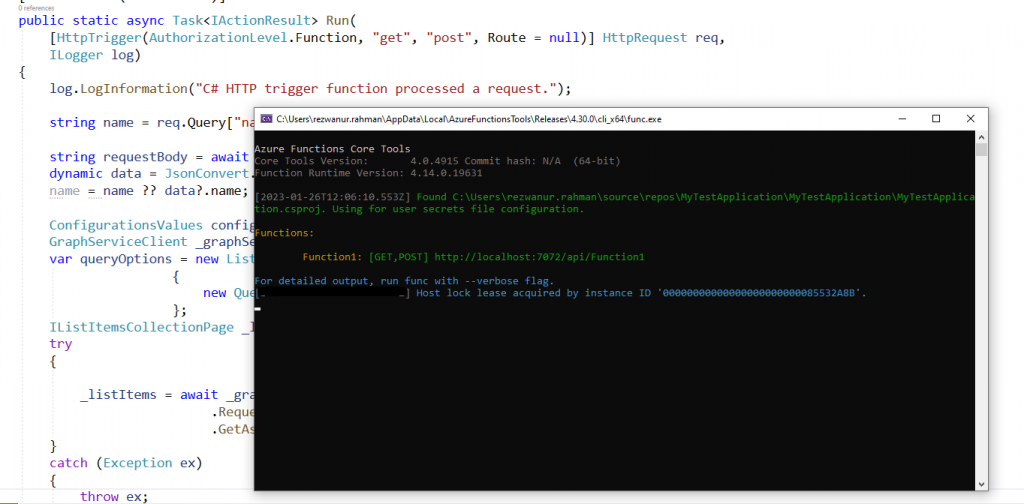
Go to browser and enter URL: localhost:7072/api/Function1
Good Luck!