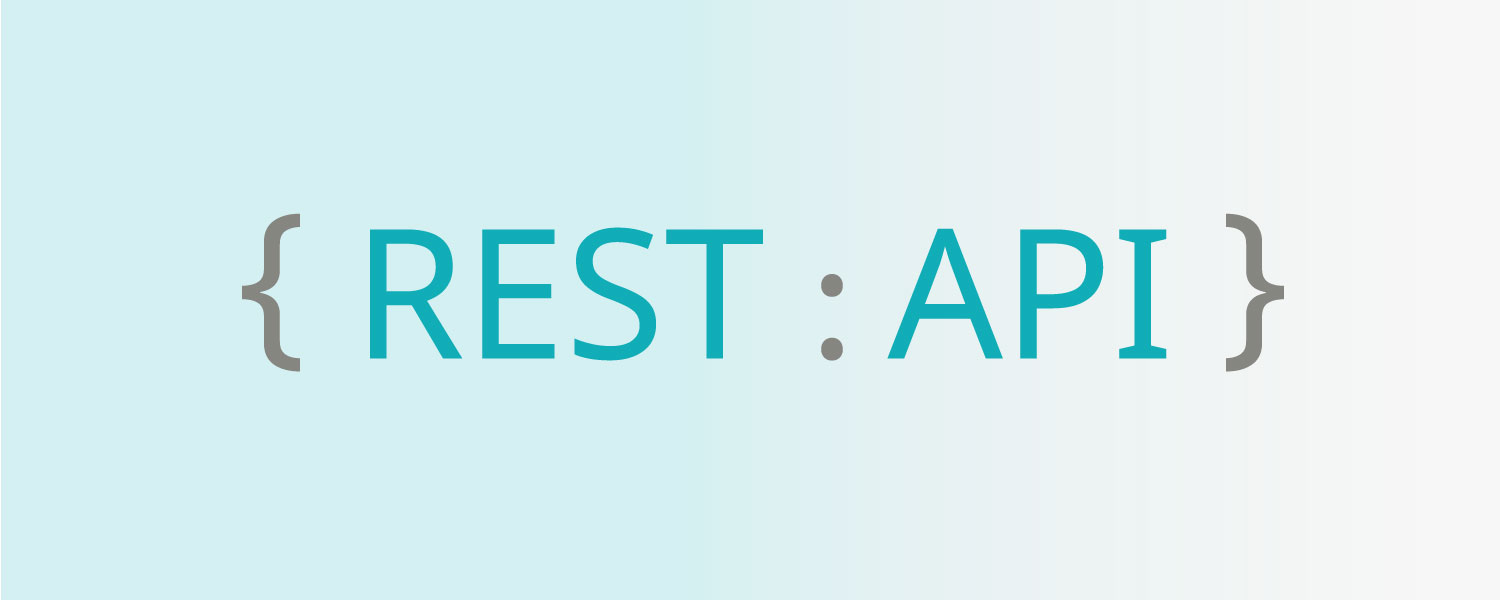
This blog will teach you how to use the JavaScript REST API to upload files to the SharePoint Document library.
Let’s get started.
Upload any File to Document Library using REST API in just 3 simple steps
STEP 1
Specify the type of a file in HTML, here I have specified DOCX and PDF
<div style="margin-top: 10%;">
<input id = "attachment" type = "file" multiple accept = ".DOCX,.docx,.txt,.TXT,.PDF,.pdf" />
<br/>
<div>
<button id = "addFileButton" type = "button" class="btn btn-primary" onclick = "uploadDocument()">Upload</button>
</div>
</div>
STEP 2
After selecting the document from the box that appears, click the upload button to invoke the uploadDocument() function.
When the user clicks on the button without first selecting a file, a warning alert appears.
function uploadDocument() {
var files = $("#attachment")[0].files;
if (files.length > 0) {
fileName = files[0].name;
var webUrl = _spPageContextInfo.webAbsoluteUrl;
var documentLibrary = "DemoLibrary";
var targetUrl = _spPageContextInfo.webServerRelativeUrl + "/" + documentLibrary;
// Construct the Endpoint
var url = webUrl + "/_api/Web/GetFolderByServerRelativeUrl(@target)/Files/add(overwrite=true, url='" + fileName + "')?@target='" + targetUrl + "'&$expand=ListItemAllFields";
uploadFileToFolder(files[0], url, function(data) {
var file = data.d;
DocFileName = file.Name;
var updateObject = {
__metadata: {
type: file.ListItemAllFields.__metadata.type
},
FileLeafRef: DocFileName //FileLeafRef --> Internal Name for Name Column
};
alert("File uploaded successfully!");
}, function(data) {
alert("File uploading failed");
});
} else {
alert("Kindly select a file to upload.!");
}
STEP 3
// Get the local file as an array buffer.
function getFileBuffer(uploadFile) {
var deferred = jQuery.Deferred();
var reader = new FileReader();
reader.onloadend = function(e) {
deferred.resolve(e.target.result);
}
reader.onerror = function(e) {
deferred.reject(e.target.error);
}
reader.readAsArrayBuffer(uploadFile);
return deferred.promise();
}
The file is uploaded to the document folder using an array buffer (local file Meta Data) and the POST method.
function uploadFileToFolder(fileObj, url, success, failure) {
var apiUrl = url;
// Initiate method calls using jQuery promises.
// Get the local file as an array buffer.
var getFile = getFileBuffer(fileObj);
// Add the file to the SharePoint folder.
getFile.done(function(arrayBuffer) {
$.ajax({
url: apiUrl,//File Collection Endpoint
type: "POST",
data: arrayBuffer,
processData: false,
async: false,
headers: {
"accept": "application/json;odata=verbose",
"X-RequestDigest": jQuery("#__REQUESTDIGEST").val(),
},
success: function(data) {
success(data);
},
error: function(data) {
success(data);
}
});
});
}
You can download the code from Github. Thanks for reading! See you in next blog!