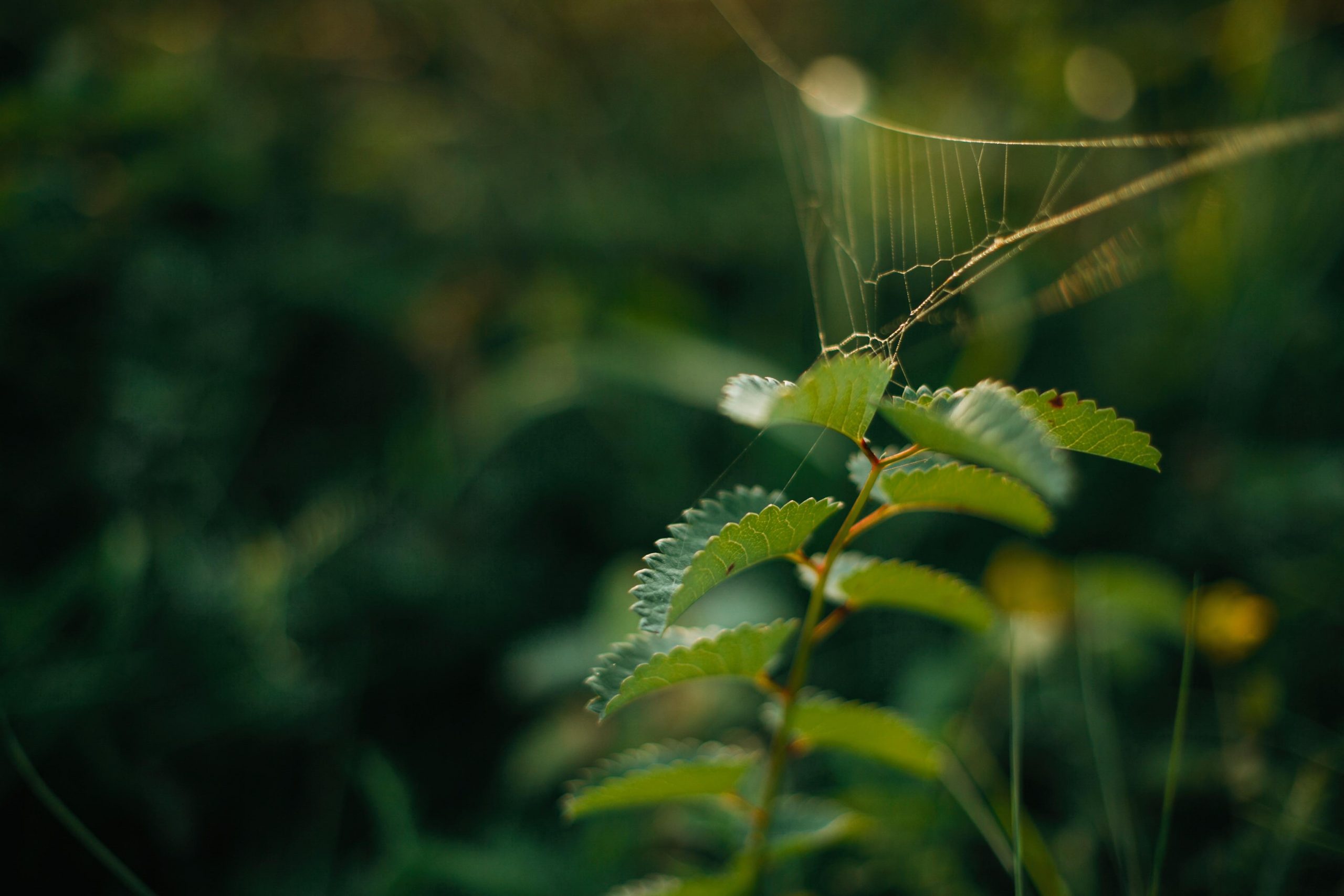
Welcome back to my blog!
Today we will discuss something awesome, MGT. The Microsoft Graph Toolkit (MGT) is an invaluable tool for me as a developer, especially when I need to integrate Microsoft Graph functionalities into my web applications. It offers a variety of reusable web components and authentication helpers, significantly streamlining my work with the Microsoft Graph API. In this blog, I’ll explore the technical aspects of MGT in depth, share advanced tips and tricks, and highlight its limitations.
Let’s check the key features, and limitations of MGT.
Key Feature | Description | Limitations |
---|---|---|
Reusable Web Components | Pre-built components for common Microsoft Graph functionalities like login, calendar, tasks. | Limited customization in terms of design and functionality. |
Authentication Providers | Simplifies authentication with Microsoft Graph using providers like MSAL. | Tied mainly to Microsoft identity platform. |
Microsoft Teams Integration | Components tailored for Microsoft Teams, such as Teams channel picker. | Specific to Microsoft Teams; not applicable for other platforms. |
Automatic Token Management | Handles token acquisition and renewal seamlessly. | Relies on proper configuration and scopes. |
Adaptive Cards Integration | Supports rendering of adaptive cards within MGT components. | Requires knowledge of Adaptive Card schema. |
Cross-Framework Compatibility | Works with various web frameworks like React, Angular, Vue.js. | Might require additional wrappers or configuration. |
Customizable Templates | Allows custom templates for rendering data. | Customization can be complex for intricate data structures. |
Event Handling | MGT components emit custom events for enhanced interactivity. | Requires understanding of event-driven programming. |
Graph Toolkit Playground | An online tool to experiment with MGT components and see live previews. | Limited to experimentation; not for production use. |
Responsive Design | Components are responsive and adapt to different screen sizes. | May not fit all design requirements or specific layouts. |
If you are already using Microsoft Graph Toolkit, then you are AWESOME! But those, who are new, lets discuss about its prerequisites, installation and configuration!
Prerequisites
To install or use MGT, you need to fill up the requirements:
- Node.js and npm installed.
- A Microsoft Azure account.
- A registered application in Azure with permission to access Microsoft Graph.
Installation
Install MGT via npm:
npm install @microsoft/mgt
Basic Setup
In your HTML file, include the toolkit components:
Key Web Components and Usage
1. Login Component
The mgt-login
component provides a simple way to integrate the Microsoft login.
2. People Picker
The mgt-people-picker
component lets users search and select people from Microsoft Graph.
3. Agenda
The mgt-agenda
component displays events from a user’s calendar.
4. Person Component
The mgt-person
component displays a person or contact by their photo, name, and/or email address.
5. Todo Component
The mgt-todo
component displays and allows interaction with tasks from Microsoft To Do
6. Get Component
The mgt-get
component allows you to make GET requests to the Microsoft Graph API directly in your HTML.
7. Tasks Component
The mgt-tasks
manages tasks from Microsoft Planner or Microsoft To Do.
8. File Component
The mgt-file
represents a file or folder.
9. File List Component
The mgt-file-list
displays a list of files or folders.
10. People Component
The mgt-people
displays a group of people or contacts.
11. Person Card Component
The mgt-person-card
shows more detailed information about a person.
12. Picker Component
The mgt-picker
renders a dropdown to select a single resource.
13. Search Box Component
The mgt-search-box
provides a search interface.
14. Search Results Component
The mgt-search-results
displays search results.
15. Taxonomy Picker Component
The mgt-taxonomy-picker
queries and displays terms from Microsoft Graph API.
16. Teams Channel Component
The mgt-teams-channel-picker
allows selection of Microsoft Teams channels.
17. Theme Toggle Component
The mgt-theme-toggle
switches between light and dark themes.
Authentication Providers
To use MGT components that require access to Microsoft Graph, you must set up an authentication provider. Here’s how to set up MSAL provider:
import {Providers, MsalProvider} from '@microsoft/mgt';
Providers.globalProvider = new MsalProvider({clientId: 'YOUR_CLIENT_ID'});
Customization and Theming
MGT components are customizable. You can modify their appearance using CSS variables.
Example: Customizing the mgt-login
component.
mgt-login {
--background-color: #004578;
--color: white;
--font-size: 16px;
}
Tips and Tricks
Some tips and tricks for new learners!
- Lazy Loading: Load components only when needed to improve performance.
- Caching Strategies: Utilize caching to reduce the number of calls to Microsoft Graph.
- Event Handlers: Leverage the events provided by MGT components for better control and interactivity.
- Debugging: Use browser dev tools to inspect and debug MGT components.
- Use Batch Requests: Group multiple Graph API requests into a single call using
mgt-get
.
Advanced Scenarios
Integrating with Frameworks
Integrating MGT with frameworks like React or Angular is straightforward. Here’s an example with React:
import React from 'react';
import { PeoplePicker } from '@microsoft/mgt-react';
function App() {
return (
);
}
export default App;
Custom Components
You can create custom components using MGT as a base. This allows for highly tailored features specific to your application.
Microsoft Graph Toolkit offers a powerful, easy-to-use set of tools and components for integrating Microsoft Graph functionality into web applications. By understanding its core components, authentication methods, and customization capabilities, developers can enhance their applications with rich Microsoft 365 integrations.